在nodejs中使用express来搭建框架可以说是非常的简单方便,但是一般默认创建的都是http服务器,也就是只能通过http协议进行访问。如今https已经是发展趋势,我们应该顺应时代的潮流。这里,将记录下这个的配置过程。
生成证书文件
先进入到项目的目录。
cd /alidata/service/node.js/server/im/
1. 生成私钥key文件
openssl genrsa 1024 > private.pem
2.通过上面生成的私钥文件生成CSR证书签名
openssl req -new -key private.pem -out csr.pem
这一步,需要输入一些证书的相关信息。比如国家,公司名,邮箱等。
参照如下:
Country Name (2 letter code)[XX]:cn--------国家
State or Province Name (full name)[]:ning---------省份
Locality Name (eg, city) [DefaultCity]:ning--------------地区名字
Organization Name (eg, company)[Default Company Ltd]:ning------公司名
Organizational Unit Name (eg,section) []:ning-----部门
Common Name (eg, your name or yourserver's hostname) []:wukui----CA主机名
Email Address []:---------邮箱
A challenge password []:-----------证书请求密钥,CA读取证书的时候需要输入密码
An optional company name[]:-----------公司名称,CA读取证书的时候需要输入名称
3.通过上述私钥文件和CSR证书签名生成证书文件
openssl x509 -req -days 365 -in csr.pem -signkey private.pem -out file.crt
创建成功后,提示 Signature ok 以及证书的一些信息。
到此,三个文件已经生成。ls一下,就可以看到文件了。
程序中使用https
编辑 index.js
var express = require('express');
var app = express();
var path = require('path');
var fs = require('fs');
// node.js自带的http、https模块
var http = require('https');
var https = require('https');
var httpServer = http.createServer(app);
// 根据项目的路径导入生成的证书文件
var privateKey = fs.readFileSync(path.join(__dirname, './cer/private.pem'), 'utf8');
var certificate = fs.readFileSync(path.join(__dirname, './cer/file.crt'), 'utf8');
var credentials = {key:privateKey, cert:certificate};
var httpsServer = https.createServer(credentials, app);
const PORT = 3000;
const SSLPORT = 3080;
// 创建http服务器
httpServer.listen(PORT, function() {
console.log('HTTP Server is running on: http://localhost:%s', PORT);
});
// 创建https服务器
httpsServer.listen(SSLPORT, function() {
console.log('HTTPS Server is running on: https://localhost:%s', SSLPORT);
});
app.get('/', function(req, res){
if(req.protocol === 'https') {
res.status(200).send('This is https visit!');
}
else {
res.status(200).send('This is http visit!');
}
});
运行服务:
node index.js
你会发现会输出:
HTTP Server is running on: http://localhost:3000
HTTPS Server is running on: https://localhost:3080
然后,在浏览器中访问:https://112.xx.xxx.xxx:3080/
服务运行在阿里云上,上边的ip地址是阿里云服务器的地址。客户端访问是本地电脑浏览器。比如chrome。
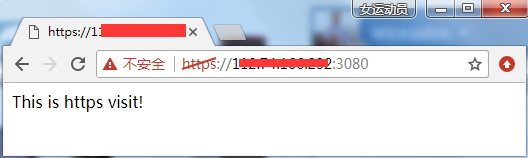
因为这个证书是我们自建的,没有经过第三方机构验证,所以会出现警告的提示。F12在控制台的Security下可以看到证书的一些信息。
socket.io使用https相关
https://stackoverflow.com/questions/31156884/how-to-use-https-on-node-js-using-express-socket-io
https://stackoverflow.com/questions/27765638/nodejs-socket-io-https
https://stackoverflow.com/questions/17285180/use-both-http-and-https-for-socket-io
https://chrislarson.me/blog/ssl-nodejs-express-and-socketio/