skynet 最早就能提供 socket 服务。后来,也能提供 websocket 服务。这里,接着之前的代码,构建一个 websocket 服务。
https://github.com/cloudwu/skynet/blob/master/examples/simplewebsocket.lua
上边是官方 websocket 的一个示例。
开始
以官方为借鉴,这里先创建一个 websocket.lua 文件。完整的路径是 www/websocket/service/connect/websocket.lua
。
代码如下
websocket.lua
local skynet = require "skynet"
local socket = require "skynet.socket"
local websocket = require "http.websocket"
local console = require 'utils/console'
local cmd = {}
local handler = {}
local clients = {}
local is_run = true
-- 接受 skynet.newservice 传递过来的参数
local params = { ... }
-- 通过 id 获取 client 信息
function cmd.getClientById(id)
for _, client in pairs(clients) do
if (client.fd == id) then
return client
end
end
return nil
end
-- 通过 connect code 获取 client 信息
function cmd.getClientByCode(code)
if clients[code] == nil then return end
return clients[code]
end
local function closeConnect(connect_code)
if clients[connect_code] == nil then return end
console.log(string.format('断开连接 addr:%s fd:%s', clients[connect_code]['addr'], clients[connect_code]['fd']))
-- 清理退出相关逻辑(用户退出房间,用户退出系统)
-- 清理 websocket
local fd = clients[connect_code]['fd']
clients[connect_code] = nil
websocket.close(fd)
end
function handler.connect(id)
console.log('ws connect from: ' .. tostring(id))
local client = cmd.getClientById(id)
if client ~= nil then
closeConnect(client.connect_code)
end
end
function handler.handshake(id, header, url)
local addr = websocket.addrinfo(id)
console.log(string.format('ws handshake from: %s url: %s addr: %s', tostring(id), url, addr))
-- console.log('------ header ------')
-- for k, v in pairs(header) do
-- console.log(string.format('%s %s', k, v))
-- end
-- console.log('------------')
local client = {}
client.connect_code = console.uuid()
client.fd = id
client.addr = addr
client.time = os.time() + 12
clients[client.connect_code] = client
end
function handler.message(id, msg, msg_type)
assert(msg_type == 'binary' or msg_type == 'text')
websocket.write(id, msg)
-- 维持心跳时间(os.time 时间戳秒)
local client = cmd.getClientById(id)
if client ~= nil then
client.time = os.time() + 12
end
end
function handler.ping(id)
console.log('ws ping from: ' .. tostring(id))
end
function handler.pong(id)
console.log('ws pong from: ' .. tostring(id))
end
function handler.close(id, code, reason)
console.log(string.format('ws close from: %s %s %s', tostring(id), code, reason))
end
function handler.error(id)
console.log('ws error from: ' .. tostring(id))
end
local function websocketAccept(id, protocol, addr)
local ok, err = websocket.accept(id, handler, protocol, addr)
if not ok then
console.log('dispatch error' .. err)
end
end
-- 构建心跳
local function startHeartbeat()
while true do
-- 阻塞 10 秒
skynet.sleep(1000)
if not is_run then
break
end
if next(clients) == nil then
goto ContinueHeartbeat
end
local time = os.time()
for connect_code, client in pairs(clients) do
print(connect_code, client.fd)
if client.time <= time then
closeConnect(connect_code)
end
end
::ContinueHeartbeat::
end
end
local function start(...)
local protocol, port = ...
local id = socket.listen('0.0.0.0', port)
socket.start(id, function(id, addr)
console.log(string.format('accept client socket_id: %s addr:%s', id , addr))
websocketAccept(id, protocol, addr)
end)
-- 启动一个新的携程,来处理心跳
skynet.fork(startHeartbeat)
console.log(string.format('Listen weboscket protocol: %s port: %s', protocol, port))
end
skynet.start(function()
start(table.unpack(params))
end)
之所以放在 connect 中,也许某一天,不想用 websocket,而是用 socket 或两者都用的时候方便去对接。
中间加了一个 console 类和 serpent,也给补上。
utils/console.lua
local serpent = require 'lualib/serpent'
local console = {}
function console.log(msg)
print(serpent.block(msg))
end
function console.uuid()
local seed = { 'e', '1', '2', '3', '4', '5', '6', '7', '8', '9', 'a', 'b', 'c', 'd', 'e', 'f' }
local tb = {}
for i = 1, 32 do
table.insert(tb, seed[math.random(1, 16)])
end
local sid = table.concat(tb)
return string.format('%s-%s-%s-%s-%s',
string.sub(sid, 1, 8),
string.sub(sid, 9, 12),
string.sub(sid, 13, 16),
string.sub(sid, 17, 20),
string.sub(sid, 21, 32)
)
end
return console
lualib/serpent.lua
local n, v = "serpent", "0.30" -- (C) 2012-17 Paul Kulchenko; MIT License
local c, d = "Paul Kulchenko", "Lua serializer and pretty printer"
local snum = {[tostring(1/0)]='1/0 --[[math.huge]]',[tostring(-1/0)]='-1/0 --[[-math.huge]]',[tostring(0/0)]='0/0'}
local badtype = {thread = true, userdata = true, cdata = true}
local getmetatable = debug and debug.getmetatable or getmetatable
local pairs = function(t) return next, t end -- avoid using __pairs in Lua 5.2+
local keyword, globals, G = {}, {}, (_G or _ENV)
for _,k in ipairs({'and', 'break', 'do', 'else', 'elseif', 'end', 'false',
'for', 'function', 'goto', 'if', 'in', 'local', 'nil', 'not', 'or', 'repeat',
'return', 'then', 'true', 'until', 'while'}) do keyword[k] = true end
for k,v in pairs(G) do globals[v] = k end -- build func to name mapping
for _,g in ipairs({'coroutine', 'debug', 'io', 'math', 'string', 'table', 'os'}) do
for k,v in pairs(type(G[g]) == 'table' and G[g] or {}) do globals[v] = g..'.'..k end end
local function s(t, opts)
local name, indent, fatal, maxnum = opts.name, opts.indent, opts.fatal, opts.maxnum
local sparse, custom, huge = opts.sparse, opts.custom, not opts.nohuge
local space, maxl = (opts.compact and '' or ' '), (opts.maxlevel or math.huge)
local maxlen, metatostring = tonumber(opts.maxlength), opts.metatostring
local iname, comm = '_'..(name or ''), opts.comment and (tonumber(opts.comment) or math.huge)
local numformat = opts.numformat or "%.17g"
local seen, sref, syms, symn = {}, {'local '..iname..'={}'}, {}, 0
local function gensym(val) return '_'..(tostring(tostring(val)):gsub("[^%w]",""):gsub("(%d%w+)",
-- tostring(val) is needed because __tostring may return a non-string value
function(s) if not syms[s] then symn = symn+1; syms[s] = symn end return tostring(syms[s]) end)) end
local function safestr(s) return type(s) == "number" and tostring(huge and snum[tostring(s)] or numformat:format(s))
or type(s) ~= "string" and tostring(s) -- escape NEWLINE/010 and EOF/026
or ("%q"):format(s):gsub("\010","n"):gsub("\026","\\026") end
local function comment(s,l) return comm and (l or 0) < comm and ' --[['..select(2, pcall(tostring, s))..']]' or '' end
local function globerr(s,l) return globals[s] and globals[s]..comment(s,l) or not fatal
and safestr(select(2, pcall(tostring, s))) or error("Can't serialize "..tostring(s)) end
local function safename(path, name) -- generates foo.bar, foo[3], or foo['b a r']
local n = name == nil and '' or name
local plain = type(n) == "string" and n:match("^[%l%u_][%w_]*$") and not keyword[n]
local safe = plain and n or '['..safestr(n)..']'
return (path or '')..(plain and path and '.' or '')..safe, safe end
local alphanumsort = type(opts.sortkeys) == 'function' and opts.sortkeys or function(k, o, n) -- k=keys, o=originaltable, n=padding
local maxn, to = tonumber(n) or 12, {number = 'a', string = 'b'}
local function padnum(d) return ("%0"..tostring(maxn).."d"):format(tonumber(d)) end
table.sort(k, function(a,b)
-- sort numeric keys first: k[key] is not nil for numerical keys
return (k[a] ~= nil and 0 or to[type(a)] or 'z')..(tostring(a):gsub("%d+",padnum))
< (k[b] ~= nil and 0 or to[type(b)] or 'z')..(tostring(b):gsub("%d+",padnum)) end) end
local function val2str(t, name, indent, insref, path, plainindex, level)
local ttype, level, mt = type(t), (level or 0), getmetatable(t)
local spath, sname = safename(path, name)
local tag = plainindex and
((type(name) == "number") and '' or name..space..'='..space) or
(name ~= nil and sname..space..'='..space or '')
if seen[t] then -- already seen this element
sref[#sref+1] = spath..space..'='..space..seen[t]
return tag..'nil'..comment('ref', level) end
-- protect from those cases where __tostring may fail
if type(mt) == 'table' then
local to, tr = pcall(function() return mt.__tostring(t) end)
local so, sr = pcall(function() return mt.__serialize(t) end)
if (opts.metatostring ~= false and to or so) then -- knows how to serialize itself
seen[t] = insref or spath
t = so and sr or tr
ttype = type(t)
end -- new value falls through to be serialized
end
if ttype == "table" then
if level >= maxl then return tag..'{}'..comment('maxlvl', level) end
seen[t] = insref or spath
if next(t) == nil then return tag..'{}'..comment(t, level) end -- table empty
if maxlen and maxlen < 0 then return tag..'{}'..comment('maxlen', level) end
local maxn, o, out = math.min(#t, maxnum or #t), {}, {}
for key = 1, maxn do o[key] = key end
if not maxnum or #o < maxnum then
local n = #o -- n = n + 1; o[n] is much faster than o[#o+1] on large tables
for key in pairs(t) do if o[key] ~= key then n = n + 1; o[n] = key end end end
if maxnum and #o > maxnum then o[maxnum+1] = nil end
if opts.sortkeys and #o > maxn then alphanumsort(o, t, opts.sortkeys) end
local sparse = sparse and #o > maxn -- disable sparsness if only numeric keys (shorter output)
for n, key in ipairs(o) do
local value, ktype, plainindex = t[key], type(key), n <= maxn and not sparse
if opts.valignore and opts.valignore[value] -- skip ignored values; do nothing
or opts.keyallow and not opts.keyallow[key]
or opts.keyignore and opts.keyignore[key]
or opts.valtypeignore and opts.valtypeignore[type(value)] -- skipping ignored value types
or sparse and value == nil then -- skipping nils; do nothing
elseif ktype == 'table' or ktype == 'function' or badtype[ktype] then
if not seen[key] and not globals[key] then
sref[#sref+1] = 'placeholder'
local sname = safename(iname, gensym(key)) -- iname is table for local variables
sref[#sref] = val2str(key,sname,indent,sname,iname,true) end
sref[#sref+1] = 'placeholder'
local path = seen[t]..'['..tostring(seen[key] or globals[key] or gensym(key))..']'
sref[#sref] = path..space..'='..space..tostring(seen[value] or val2str(value,nil,indent,path))
else
out[#out+1] = val2str(value,key,indent,insref,seen[t],plainindex,level+1)
if maxlen then
maxlen = maxlen - #out[#out]
if maxlen < 0 then break end
end
end
end
local prefix = string.rep(indent or '', level)
local head = indent and '{\n'..prefix..indent or '{'
local body = table.concat(out, ','..(indent and '\n'..prefix..indent or space))
local tail = indent and "\n"..prefix..'}' or '}'
return (custom and custom(tag,head,body,tail,level) or tag..head..body..tail)..comment(t, level)
elseif badtype[ttype] then
seen[t] = insref or spath
return tag..globerr(t, level)
elseif ttype == 'function' then
seen[t] = insref or spath
if opts.nocode then return tag.."function() --[[..skipped..]] end"..comment(t, level) end
local ok, res = pcall(string.dump, t)
local func = ok and "((loadstring or load)("..safestr(res)..",'@serialized'))"..comment(t, level)
return tag..(func or globerr(t, level))
else return tag..safestr(t) end -- handle all other types
end
local sepr = indent and "\n" or ";"..space
local body = val2str(t, name, indent) -- this call also populates sref
local tail = #sref>1 and table.concat(sref, sepr)..sepr or ''
local warn = opts.comment and #sref>1 and space.."--[[incomplete output with shared/self-references skipped]]" or ''
return not name and body..warn or "do local "..body..sepr..tail.."return "..name..sepr.."end"
end
local function deserialize(data, opts)
local env = (opts and opts.safe == false) and G
or setmetatable({}, {
__index = function(t,k) return t end,
__call = function(t,...) error("cannot call functions") end
})
local f, res = (loadstring or load)('return '..data, nil, nil, env)
if not f then f, res = (loadstring or load)(data, nil, nil, env) end
if not f then return f, res end
if setfenv then setfenv(f, env) end
return pcall(f)
end
local function merge(a, b) if b then for k,v in pairs(b) do a[k] = v end end; return a; end
return { _NAME = n, _COPYRIGHT = c, _DESCRIPTION = d, _VERSION = v, serialize = s,
load = deserialize,
dump = function(a, opts) return s(a, merge({name = '_', compact = true, sparse = true}, opts)) end,
line = function(a, opts) return s(a, merge({sortkeys = true, comment = true}, opts)) end,
block = function(a, opts) return s(a, merge({indent = ' ', sortkeys = true, comment = true}, opts)) end }
websocket
服务弄好了,在 main.lua
中用起来。
main.lua
…
skynet.start(function()
local port = 8091
local ws = 'ws'
skynet.newservice('service/connect/websocket', ws, port)
end)
还有, docker-compose.yaml 将 8091 端口得暴露出来。
websocket:
image: skynet:1.7
container_name: websocket
volumes:
- ./www:/www
ports:
- 8081:8081
command: /skynet/skynet /www/websocket/etc/config
代码都准备好了,那么跑起来看看。
docker-compose up
如果看到 websocket | "Listen weboscket protocol: ws port: 8081",表示服务已经起来了。
然后在 web 端,新建一个简单的 websocket.html 文件。代码如下。
<!DOCTYPE html>
<html>
<head>
<title>skynet WebSocket example</title>
</head>
<body>
<script>
var ws = new WebSocket("ws://127.0.0.1:8081");
ws.onopen = function () {
run();
setInterval(run, 5000);
};
ws.onmessage = function (ev) {};
ws.onclose = function (ev) {};
ws.onerror = function (ev) {};
function run() {
const data = { num: Math.floor(Math.random() * 1000)}
ws.send(JSON.stringify(data));
}
</script>
</body>
</html>
使用 http-server 等工具构建一个服务,跑起来测试看看。
这个时候如果在服务端看到下边这样的。
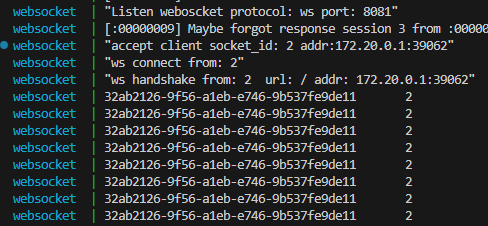
在网页端看到是这样的。
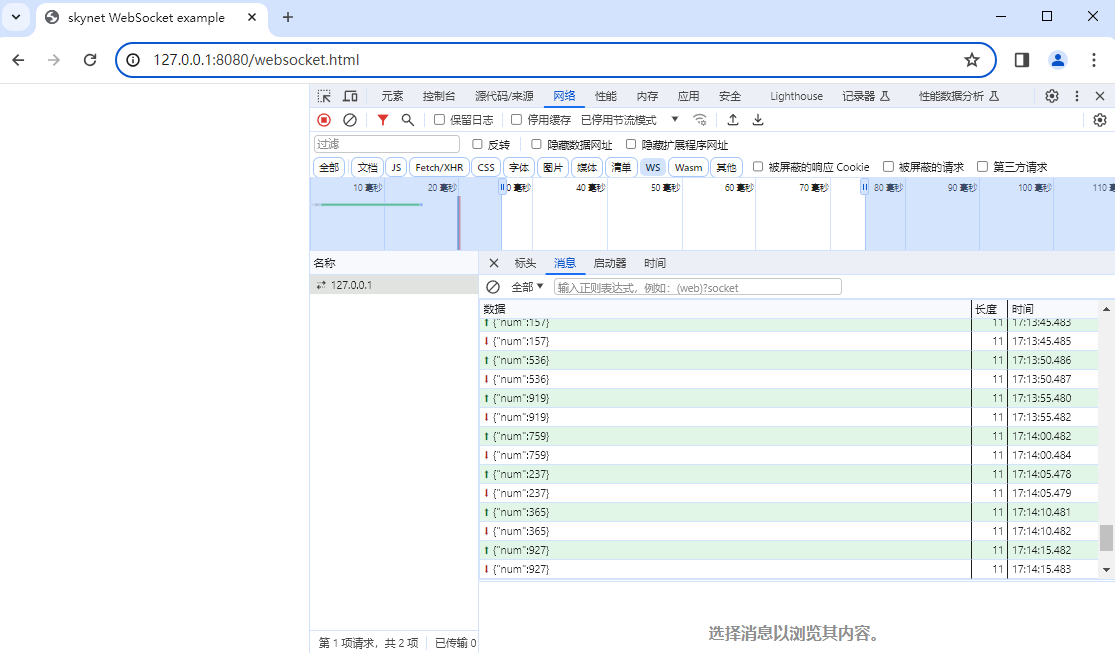
那就说明,websocket 基础联通了。然后做一下断开的测试等。